mirror of
https://github.com/freedomofpress/dangerzone.git
synced 2025-04-28 18:02:38 +02:00
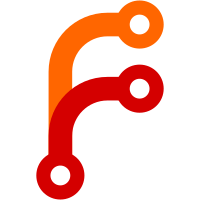
Some checks are pending
Build dev environments / Build dev-env (debian-bookworm) (push) Waiting to run
Build dev environments / Build dev-env (debian-bullseye) (push) Waiting to run
Build dev environments / Build dev-env (debian-trixie) (push) Waiting to run
Build dev environments / Build dev-env (fedora-39) (push) Waiting to run
Build dev environments / Build dev-env (fedora-40) (push) Waiting to run
Build dev environments / Build dev-env (fedora-41) (push) Waiting to run
Build dev environments / Build dev-env (ubuntu-20.04) (push) Waiting to run
Build dev environments / Build dev-env (ubuntu-22.04) (push) Waiting to run
Build dev environments / Build dev-env (ubuntu-23.10) (push) Waiting to run
Build dev environments / Build dev-env (ubuntu-24.04) (push) Waiting to run
Build dev environments / Build dev-env (ubuntu-24.10) (push) Waiting to run
Build dev environments / build-container-image (push) Waiting to run
Check branch conformity / prevent-fixup-commits (push) Waiting to run
Tests / run-lint (push) Waiting to run
Tests / build-container-image (push) Waiting to run
Tests / Download and cache Tesseract data (push) Waiting to run
Tests / windows (push) Blocked by required conditions
Tests / macOS (arch64) (push) Blocked by required conditions
Tests / macOS (x86_64) (push) Blocked by required conditions
Tests / build-deb (debian bookworm) (push) Blocked by required conditions
Tests / build-deb (debian bullseye) (push) Blocked by required conditions
Tests / build-deb (debian trixie) (push) Blocked by required conditions
Tests / build-deb (ubuntu 20.04) (push) Blocked by required conditions
Tests / build-deb (ubuntu 22.04) (push) Blocked by required conditions
Tests / build-deb (ubuntu 23.10) (push) Blocked by required conditions
Tests / build-deb (ubuntu 24.04) (push) Blocked by required conditions
Tests / build-deb (ubuntu 24.10) (push) Blocked by required conditions
Tests / install-deb (debian bookworm) (push) Blocked by required conditions
Tests / install-deb (debian bullseye) (push) Blocked by required conditions
Tests / install-deb (debian trixie) (push) Blocked by required conditions
Tests / install-deb (ubuntu 20.04) (push) Blocked by required conditions
Tests / install-deb (ubuntu 22.04) (push) Blocked by required conditions
Tests / install-deb (ubuntu 23.10) (push) Blocked by required conditions
Tests / install-deb (ubuntu 24.04) (push) Blocked by required conditions
Tests / install-deb (ubuntu 24.10) (push) Blocked by required conditions
Tests / build-install-rpm (fedora 39) (push) Blocked by required conditions
Tests / build-install-rpm (fedora 40) (push) Blocked by required conditions
Tests / build-install-rpm (fedora 41) (push) Blocked by required conditions
Tests / run tests (debian bookworm) (push) Blocked by required conditions
Tests / run tests (debian bullseye) (push) Blocked by required conditions
Tests / run tests (debian trixie) (push) Blocked by required conditions
Tests / run tests (fedora 39) (push) Blocked by required conditions
Tests / run tests (fedora 40) (push) Blocked by required conditions
Tests / run tests (fedora 41) (push) Blocked by required conditions
Tests / run tests (ubuntu 20.04) (push) Blocked by required conditions
Tests / run tests (ubuntu 22.04) (push) Blocked by required conditions
Tests / run tests (ubuntu 23.10) (push) Blocked by required conditions
Tests / run tests (ubuntu 24.04) (push) Blocked by required conditions
Tests / run tests (ubuntu 24.10) (push) Blocked by required conditions
Scan latest app and container / security-scan-container (push) Waiting to run
Scan latest app and container / security-scan-app (push) Waiting to run
121 lines
3.6 KiB
Python
121 lines
3.6 KiB
Python
import os
|
|
|
|
import pytest
|
|
from pytest_mock import MockerFixture
|
|
from pytest_subprocess import FakeProcess
|
|
|
|
from dangerzone.isolation_provider.container import (
|
|
Container,
|
|
ImageInstallationException,
|
|
ImageNotPresentException,
|
|
NotAvailableContainerTechException,
|
|
)
|
|
from dangerzone.isolation_provider.qubes import is_qubes_native_conversion
|
|
|
|
from .base import IsolationProviderTermination, IsolationProviderTest
|
|
|
|
# Run the tests in this module only if we can spawn containers.
|
|
if is_qubes_native_conversion():
|
|
pytest.skip("Qubes native conversion is enabled", allow_module_level=True)
|
|
elif os.environ.get("DUMMY_CONVERSION", False):
|
|
pytest.skip("Dummy conversion is enabled", allow_module_level=True)
|
|
|
|
|
|
@pytest.fixture
|
|
def provider() -> Container:
|
|
return Container()
|
|
|
|
|
|
class TestContainer(IsolationProviderTest):
|
|
def test_is_runtime_available_raises(
|
|
self, provider: Container, fp: FakeProcess
|
|
) -> None:
|
|
"""
|
|
NotAvailableContainerTechException should be raised when
|
|
the "podman image ls" command fails.
|
|
"""
|
|
fp.register_subprocess(
|
|
[provider.get_runtime(), "image", "ls"],
|
|
returncode=-1,
|
|
stderr="podman image ls logs",
|
|
)
|
|
with pytest.raises(NotAvailableContainerTechException):
|
|
provider.is_runtime_available()
|
|
|
|
def test_is_runtime_available_works(
|
|
self, provider: Container, fp: FakeProcess
|
|
) -> None:
|
|
"""
|
|
No exception should be raised when the "podman image ls" can return properly.
|
|
"""
|
|
fp.register_subprocess(
|
|
[provider.get_runtime(), "image", "ls"],
|
|
)
|
|
provider.is_runtime_available()
|
|
|
|
def test_install_raise_if_image_cant_be_installed(
|
|
self, mocker: MockerFixture, provider: Container, fp: FakeProcess
|
|
) -> None:
|
|
"""When an image installation fails, an exception should be raised"""
|
|
|
|
fp.register_subprocess(
|
|
[provider.get_runtime(), "image", "ls"],
|
|
)
|
|
|
|
# First check should return nothing.
|
|
fp.register_subprocess(
|
|
[
|
|
provider.get_runtime(),
|
|
"image",
|
|
"list",
|
|
"--format",
|
|
"{{.ID}}",
|
|
"dangerzone.rocks/dangerzone",
|
|
],
|
|
occurrences=2,
|
|
)
|
|
|
|
# Make podman load fail
|
|
mocker.patch("gzip.open", mocker.mock_open(read_data=""))
|
|
|
|
fp.register_subprocess(
|
|
[provider.get_runtime(), "load"],
|
|
returncode=-1,
|
|
)
|
|
|
|
with pytest.raises(ImageInstallationException):
|
|
provider.install()
|
|
|
|
def test_install_raises_if_still_not_installed(
|
|
self, mocker: MockerFixture, provider: Container, fp: FakeProcess
|
|
) -> None:
|
|
"""When an image keep being not installed, it should return False"""
|
|
|
|
fp.register_subprocess(
|
|
[provider.get_runtime(), "image", "ls"],
|
|
)
|
|
|
|
# First check should return nothing.
|
|
fp.register_subprocess(
|
|
[
|
|
provider.get_runtime(),
|
|
"image",
|
|
"list",
|
|
"--format",
|
|
"{{.ID}}",
|
|
"dangerzone.rocks/dangerzone",
|
|
],
|
|
occurrences=2,
|
|
)
|
|
|
|
# Patch gzip.open and podman load so that it works
|
|
mocker.patch("gzip.open", mocker.mock_open(read_data=""))
|
|
fp.register_subprocess(
|
|
[provider.get_runtime(), "load"],
|
|
)
|
|
with pytest.raises(ImageNotPresentException):
|
|
provider.install()
|
|
|
|
|
|
class TestContainerTermination(IsolationProviderTermination):
|
|
pass
|