mirror of
https://github.com/freedomofpress/dangerzone.git
synced 2025-04-28 18:02:38 +02:00
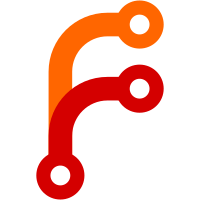
Skip the creation of the `share/container.tar` file, since it's not used anywhere. Instead, pipe our `docker/podman save` invocations to `gzip` directly, which will compress the tarfile on the fly. This saves both time and disk space.
59 lines
1.2 KiB
Python
59 lines
1.2 KiB
Python
import gzip
|
|
import os
|
|
import subprocess
|
|
|
|
|
|
def main():
|
|
print("Building container image")
|
|
subprocess.run(
|
|
[
|
|
"docker",
|
|
"build",
|
|
"container",
|
|
"--platform",
|
|
"linux/amd64",
|
|
"--tag",
|
|
"dangerzone.rocks/dangerzone",
|
|
]
|
|
)
|
|
|
|
print("Saving container image")
|
|
cmd = subprocess.Popen(
|
|
[
|
|
"docker",
|
|
"save",
|
|
"dangerzone.rocks/dangerzone",
|
|
],
|
|
stdout=subprocess.PIPE,
|
|
)
|
|
|
|
print("Compressing container image")
|
|
chunk_size = 4 << 12
|
|
with gzip.open("share/container.tar.gz", "wb") as gzip_f:
|
|
while True:
|
|
chunk = cmd.stdout.read(chunk_size)
|
|
if len(chunk) > 0:
|
|
gzip_f.write(chunk)
|
|
else:
|
|
break
|
|
|
|
cmd.wait(5)
|
|
|
|
print("Looking up the image id")
|
|
image_id = subprocess.check_output(
|
|
[
|
|
"docker",
|
|
"image",
|
|
"list",
|
|
"--format",
|
|
"{{.ID}}",
|
|
"dangerzone.rocks/dangerzone",
|
|
],
|
|
text=True,
|
|
)
|
|
with open("share/image-id.txt", "w") as f:
|
|
f.write(image_id)
|
|
|
|
|
|
if __name__ == "__main__":
|
|
main()
|