mirror of
https://github.com/freedomofpress/dangerzone.git
synced 2025-04-28 18:02:38 +02:00
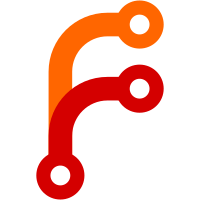
Add a fixture that returns our stock Dummy provider. Also, explicitly use a blocking Dummy provider (`DummyWait`) for a specific test case. This will prove useful when we stop using the `provider_wait` variant of our isolation providers in the next commits.
62 lines
1.7 KiB
Python
62 lines
1.7 KiB
Python
import os
|
|
import subprocess
|
|
|
|
import pytest
|
|
from pytest_mock import MockerFixture
|
|
|
|
from dangerzone.conversion import errors
|
|
from dangerzone.document import Document
|
|
from dangerzone.isolation_provider.base import IsolationProvider
|
|
from dangerzone.isolation_provider.dummy import Dummy
|
|
|
|
from .base import IsolationProviderTermination
|
|
|
|
# Run the tests in this module only if dummy conversion is enabled.
|
|
if not os.environ.get("DUMMY_CONVERSION", False):
|
|
pytest.skip("Dummy conversion is not enabled", allow_module_level=True)
|
|
|
|
|
|
class DummyWait(Dummy):
|
|
"""Dummy isolation provider that spawns a blocking process."""
|
|
|
|
def start_doc_to_pixels_proc(self, document: Document) -> subprocess.Popen:
|
|
return subprocess.Popen(
|
|
["python3"],
|
|
stdin=subprocess.PIPE,
|
|
stdout=subprocess.PIPE,
|
|
stderr=subprocess.PIPE,
|
|
start_new_session=True,
|
|
)
|
|
|
|
|
|
@pytest.fixture
|
|
def provider_wait() -> DummyWait:
|
|
return DummyWait()
|
|
|
|
|
|
@pytest.fixture
|
|
def provider() -> Dummy:
|
|
return Dummy()
|
|
|
|
|
|
class TestDummyTermination(IsolationProviderTermination):
|
|
def test_failed(
|
|
self,
|
|
provider_wait: IsolationProvider,
|
|
mocker: MockerFixture,
|
|
) -> None:
|
|
mocker.patch.object(
|
|
provider_wait,
|
|
"get_proc_exception",
|
|
return_value=errors.DocFormatUnsupported(),
|
|
)
|
|
super().test_failed(provider_wait, mocker)
|
|
|
|
def test_linger_unkillable(
|
|
self,
|
|
provider_wait: IsolationProvider,
|
|
mocker: MockerFixture,
|
|
) -> None:
|
|
# We have to spawn a blocking process here, else we can't imitate an
|
|
# "unkillable" process.
|
|
super().test_linger_unkillable(provider_wait, mocker)
|